Today I added in SMMsg suite and Viewer for MS Outlook Messages the functions to work with flags in message (PR_MESSAGE_FLAGS attribute)
The list of flags published at http://msdn.microsoft.com/en-us/library/cc839733(v=office.12).aspx
const
//The message is marked as having been read
MSGFLAG_READ = $00000001;
//The outgoing message has not been modified since the first time that it was saved; the incoming message has not been modified since it was delivered
MSGFLAG_UNMODIFIED = $00000002;
//The message is marked for sending
MSGFLAG_SUBMIT = $00000004;
//The message is still being composed. It is saved, but has not been sent
MSGFLAG_UNSENT = $00000008;
//The message has at least one attachment
MSGFLAG_HASATTACH = $00000010;
//The messaging user sending was the messaging user receiving the message
MSGFLAG_FROMME = $00000020;
//The message is an associated message of a folder
MSGFLAG_ASSOCIATED = $00000040;
//The message includes a request for a resend operation with a nondelivery report
MSGFLAG_RESEND = $00000080;
//A read report needs to be sent for the message.
MSGFLAG_RN_PENDING = $00000100;
//A nonread report needs to be sent for the message
MSGFLAG_NRN_PENDING = $00000200;
//The incoming message arrived over an X.400 link
MSGFLAG_ORIGIN_X400 = $00000400;
//The incoming message arrived over the Internet
MSGFLAG_ORIGIN_INTERNET = $00000800;
//The incoming message arrived over an external link other than X.400 or the Internet
MSGFLAG_ORIGIN_MISC_EXT = $00001000;
Now it looks so:
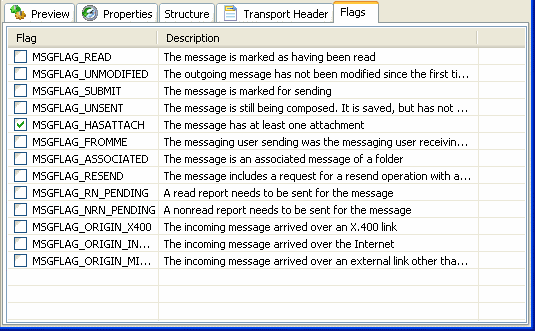